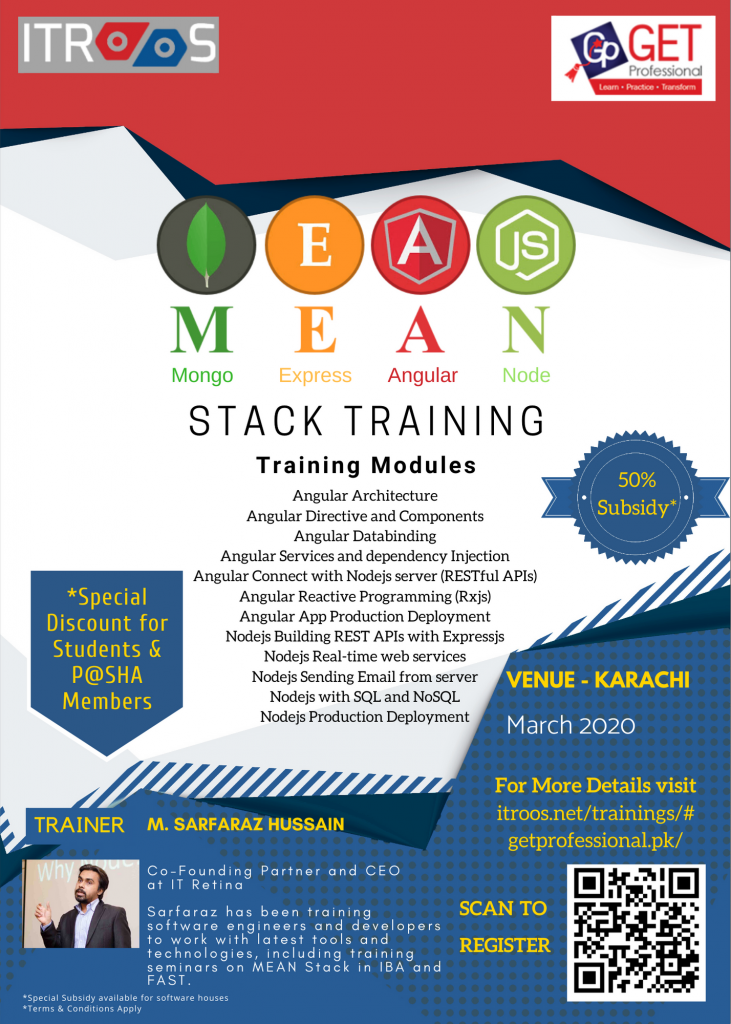
About Training & Trainer
Introduction
Learn to create modern, scalable and high-speed Web Applications with Angular (formerly named Angular 2, now just “Angular”) and NodeJS + Express + MongoDB.
MEAN stack (MongoDB, Express, Angular, and Node.js) offers you, speed, ease of development, highly reactive, awesome support for asynchronous operations, great scalability, robust, and maintainable web applications and SPAs (single-page applications), which does not require entirely refreshing a web page for every server request like most traditional web applications do.
Importance
The major benefit of the MEAN stack is that it’s extremely quick to prototype with. Node.js allows you to use Javascript on the backend as well as the frontend which can save you from having to learn a separate language. In addition, the NoSQL nature of MongoDB allows you to quickly change and alter the data layer without having to worry about migrations, which is a very valuable attribute when you’re trying to build a product without clear specifications.
Output and Impact of this training
This training follows a hands-on approach, which means that the whole course is structured around one big application and the different concepts will be explained in detail as they are introduced in this application.
Specifically, you will learn how to set up a NodeJS + Express + MongoDB + Angular. Use NodeJS and Express for creating backend routes. Build reusable Components in Angular and create a reactive User Experience with the Tools provided by Angular. Use MongoDB with Mongoose to interact with Data on the Backend and much more.
Trainers
SARFARAZ HUSSAIN
Sarfaraz is the Co-Founding Partner and CEO at IT Retina, providing enterprise services, building web and mobile solutions. He is dedicated to maintain up-to-date industry knowledge and IT skill. He has developed several web and mobile applications throughout years with expertise in Javascript, Angular, React, Node JS and web technologies revolving around these as well as Native technologies like iOS and android.
Sarfaraz has been training software engineers and developers to work with latest tools and technologies, including training seminars on MEAN Stack in IBA and FAST.
Course Outline
Angular: Part 1
- Installing and setting up Angular Project
- Understanding the Folder Structure
- Understanding Angular Components
- Creating New Components
- Understanding the Role of AppModule and Component Declaration
- Using Custom Components
- Creating Components with the CLI & Nesting Components
- Working With Components Templates
- Working with Components Styles
- Fully Understand the Component Selector
- What is Databinding?
- String Interpolation
- Property Binding
- Property Binding vs String Interpolation
- Event Binding
- Bindable Properties and Events
- Listening to Events
- Passing and Using Data with Event Binding
- FormsModule importance for Two-Way-Binding
- Understanding Two-Way-Databinding
- Understanding Directives
- Diving Into Structural Directives
- Using ngIf to output Data Conditionally
- Enhancing ngIf with an Else condition
- Styling Elements Dynamically with ngStyle
- Applying CSS Classes Dynamically with ngClass
- Creating Lists with ngFor
- Getting User Input
- Installing and setting up Angular Material UI Framework
- Adding a Toolbar
- Creating and displaying Posts
- Creating Posts with Property & Event Binding
- Creating a Post Model
Angular: Part 2
- Using Pipes to Transform Output
- Handeling Forms in Angular Application
- Adding Forms
- Creating the “edit” Form
- Observables
- Building and Using Custom Observable from Scratch
- Using Services and Dependency Injection
- Understanding the Hierarchical Inector
- Using Services for Cross-Component Communication
- Creating a Data Service
- Adding the Node Backend
- Fetching Posts from Backend
- Using the Angular HTTP Client
- Calling REST APIs
- Getting Posts from Post-Create to Post-List
- Understanding CORS
- Adding the POST Backend Point
- Setting up and Loading Routes
- Navigating with Router Links
- Understanding Navigation Paths
- Styling Active Router LInks
- Navigating Programmatically
- Passing Parameters to Routes
- Fetching Route Parameters
- Angular Animations
- Adding Offline Capabilities with Service Workers
- Using Angular Modules and Optimizing Apps
- Deploying and Angular Application
- Understanding Angular Error Messages
- Debugging Code in the Browser Using Sourcemaps
- Using Augury to Dive into Angular Apps
- Adding Loading Spinners
- Angular Universal (Rendering Angular at Server Side)
- Unit Testing in Angular Applications
Nodejs
- Installing Node.js
- Using Node.js to execute scripts
- Creating a project
- The package .JSON configuration file
- Global vs. local package installation
- Understanding NPM scripts
- Modularization
- The Common JS
- Defining modules with exports
- Modules are singletons
- Creating a package
- What is a RESTful API?
- Adding the Express Framework
- Adding Angular
Expressjs
- The model-view-controller pattern
- Building a front-end controller
- Defining routes
- Creating GET/PUT/POST/DELETE routes